Guestkey Touch Free SDK
This is the documentation for the Guestkey Touch Free SDK for Android and iOS. In order to use the SDKs, the 3rd party will be provided with:
Login credentials for this website to view API keys and API documentation.
Tenant IDs for the Tenants to which the 3rd party has access. Tenants are hotels/properties within which lock IDs will be unique.
Sandbox Tenant ID. A tenant for testing the integration. Will function the same as a normal Tenant, but will always return a Key payload that is pre-installed on the test locks.
An AAR file to import into your android project and an xcframework file to import into your iOS project
A test lock.
IMPORTANT: API keys are intended for use on private 3rd party servers and must be stored securely. They should never be shared publicly or stored or used in anyway on mobile or other publicly accessible devices.
Android requirements:
Min API level 24
Following permissions in manifest and runtime:
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.INTERNET" />Following gradle (or equivalent) imports:
implementation(files("libs/GuestkeySDK.aar"))
implementation("com.github.kittinunf.fuel:fuel:2.3.0")
implementation("com.google.code.gson:gson:2.10.1")
implementation("com.juul.able:core:0.8.0")
iOS requirements
iOS 15.0
The following diagram shows how to integrate the full solution with your mobile application and B2B servers:
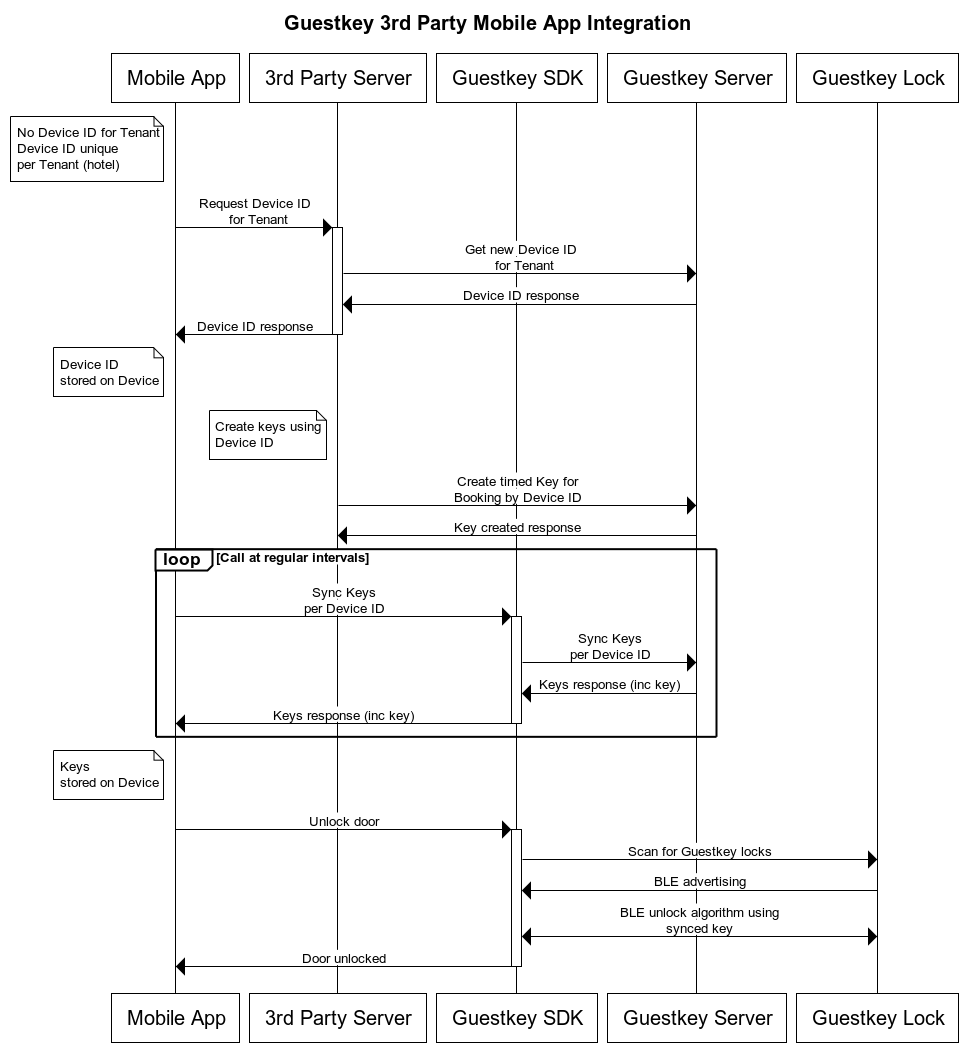
Steps:
The 3rd party server must register the Guest's Device for each Tenant required.
The Device ID(s) must be stored securely on the Guest's mobile device. It must be delivered to the mobile device via a secure mechanism and not shared with other person(s) or devices.
The 3rd party server can then create keys using the Device ID and Tenant ID. The ID of the created key is returned in the location header of the response.
On the Guest mobile device, the Sync Keys SDK function should be called at regular intervals (eg onResume, when back online after loss of connection and in background every x minutes) or if key is not present when attempting to unlock:
Android
The code uses Kotlin coroutines and runs on the
Dispatchers.IO
dispatcher (on a background thread). Example usage (for Java see below):lifecycleScope.launch {
val result = com.guestkey.sdk.syncKeys(context, tenantId, deviceId)
}
Result type:
data class Result(val success: Boolean = false, val error: String? = null)
iOS
let guestkey = GuestkeyClient()
let result = await guestkey.syncKeys(tenantId: tenantId, deviceId: deviceId)
...do something with result
The SDK will store the keys in the Device local storage for android or keychain for iOS.
When the Guest wants to unlock a lock, the Unlock SDK function should be called:
Android
Bluetooth code on Android should be run on the main thread to avoid errors. Example usage (for Java see below):
viewModelScope.launch {
val result = com.guestkey.sdk.unlock(context, tenantId)
}
Result type:
data class Result(val success: Boolean = false, val error: String? = null)
iOS
let guestkey = GuestkeyClient()
let result = await guestkey.unlock(tenantId: tenantId)
...do something with result
Possible errors from unlock function:
"No keys"
"No device found"
"Timeout"
Keys can be revoked using this API.
Java Usage:
A Continuation abstract class is provided to allow Java code to call the Kotlin suspend functions:
UnlockKt.unlock(context, tenantId, new Continuation<Result>() {
@Override
public CoroutineContext getContext() {
return EmptyCoroutineContext.INSTANCE;
}
@Override
public void resume(Result result) {
...do something with result
}
@Override
public void resumeWithException(Throwable throwable) {
...there was an exception thrown
}
});